前回の第25回
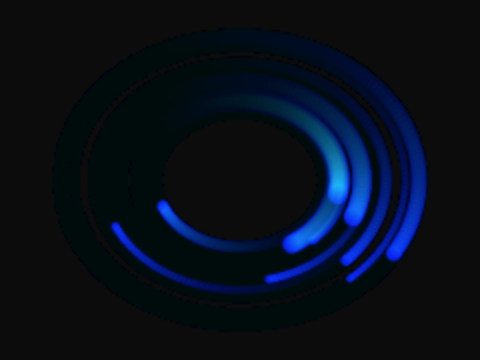
楕円軌道を速度から導く
前回のサンプルの楕円軌道は、
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
this.x = this.centerX + this.radius * Math.cos(angle);
this.y = this.centerY + this.radius * 0.8 * Math.sin(angle);
this.angle = angle;
};
このコードでインスタンスが楕円軌道を動くのは、
現行角度 += 角速度
水平座標(x) = 水平中心座標 + 半径×cos(現行角度)
垂直座標(y) = 垂直中心座標 + 半径×sin(現行角度)
上記の方程式は、
現行角度 += 角速度
水平速度 = -半径×角速度×sin(現行角度)
垂直速度 = 半径×角速度×cos(現行角度)
水平座標(x) += 水平速度
垂直座標(y) += 垂直速度
細かい説明より先に、
Particle.prototype.reset = function(x, y, radius, angle) {
// this.centerX = x;
// this.centerY = y;
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
// this.radius = radius;
this.angle = 0; // angle;
};
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
// this.x = this.centerX + this.radius * Math.cos(angle);
// this.y = this.centerY + this.radius * 0.8 * Math.sin(angle);
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
};
先に、
さらに、
クラス
function resetParticle(particle) {
// var radius = 40 + Math.random() * 50;
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
particle.reset(center.x, center.y, radius, angle);
}
楕円軌道で動くパーティクルをつくるクラス
function Particle(radius, color) {
this.initialize();
this.graphics.beginFill(color)
.drawCircle(0, 0, radius)
.endFill();
this.compositeOperation = "lighter";
}
Particle.prototype = new createjs.Shape();
Particle.prototype.reset = function(x, y, radius, angle) {
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
this.angle = 0;
};
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
};
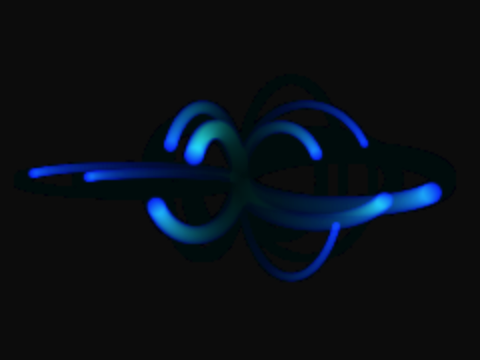
前掲コード1のクラス
var stage;
var total = 10;
var center = new createjs.Point();
var particles = [];
var fading = 0.04;
function initialize() {
var canvasElement = document.getElementById("myCanvas");
var stageWidth = canvasElement.width;
var stageHeight = canvasElement.height;
stage = new createjs.Stage(canvasElement);
stage.autoClear = false;
center.x = stageWidth / 2;
center.y = stageHeight / 2;
for(var i = 0; i < total; i++) {
var radius = 1 + Math.random() * 4;
var particle = new Particle(radius, "#0016E9");
resetParticle(particle);
particles.push(particle);
stage.addChild(particle);
}
addBackground(stageWidth, stageHeight, fading);
createjs.Ticker.timingMode = createjs.Ticker.RAF;
createjs.Ticker.addEventListener("tick", tick);
}
function resetParticle(particle) {
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
particle.reset(center.x, center.y, radius, angle);
}
function tick() {
var count = particles.length;
for(var i = 0; i < count; i++) {
var particle = particles[i];
moveParticle(particle);
}
stage.update();
}
function moveParticle(particle) {
particle.move(Math.PI / 90);
}
function addBackground(width, height, alpha) {
var background = new createjs.Shape();
background.graphics.beginFill("black")
.drawRect(0, 0, width, height)
.endFill();
stage.addChild(background);
background.alpha = alpha;
}
ランダムな楕円軌道のバランスをとる
前記の速度を求める方程式の中で
したがって、
Particle.prototype.reset = function(x, y, radius, angle) {
// this.speedX = radius * Math.cos(angle);
// this.speedY = radius * Math.sin(angle);
this.speedX = radius * (Math.random() * 2 - 1);
this.speedY = radius * (Math.random() * 2 - 1);
};
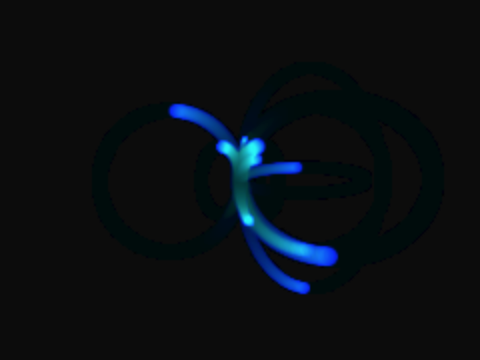
xとy方向それぞれ独立に±1の範囲のランダムな値を決めると、
100個のパーティクルを使い回して再設定する
パーティクルの数をさらに増やそう。ただ、
// Particle.prototype.reset = function(x, y, radius, angle) {
Particle.prototype.reset = function(x, y, radius, angle, lifetime) {
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
this.lifetime = lifetime;
this.angle = 0;
};
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
this.lifetime--;
};
つぎに、
そして、
var total = 100; // = 10;
function resetParticle(particle) {
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
var lifetime = Math.random()* total | 0;
// particle.reset(center.x, center.y, radius, angle);
particle.reset(center.x, center.y, radius, angle, lifetime);
}
function moveParticle(particle) {
if (particle.lifetime > 0) {
particle.move(Math.PI / 90);
} else {
resetParticle(particle);
}
}
これで、
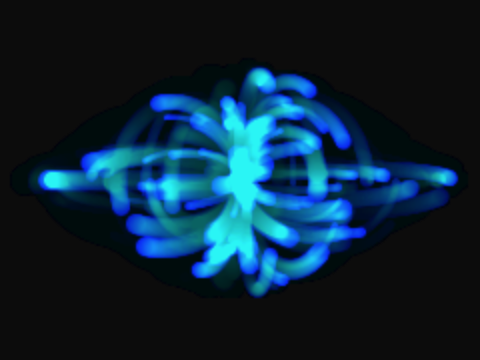
function Particle(radius, color) {
this.initialize();
this.graphics.beginFill(color)
.drawCircle(0, 0, radius)
.endFill();
this.compositeOperation = "lighter";
}
Particle.prototype = new createjs.Shape();
Particle.prototype.reset = function(x, y, radius, angle, lifetime) {
this.x = x;
this.y = y;
this.speedX = radius * Math.cos(angle);
this.speedY = radius * Math.sin(angle);
this.lifetime = lifetime;
this.angle = 0;
};
Particle.prototype.move = function(advance) {
var angle = this.angle + advance;
var velocityX = this.speedX * Math.sin(angle);
var velocityY = this.speedY * Math.cos(angle);
this.x += velocityX;
this.y += velocityY;
this.angle = angle;
this.lifetime--;
};
var stage;
var total = 100;
var center = new createjs.Point();
var particles = [];
var fading = 0.04;
function initialize() {
var canvasElement = document.getElementById("myCanvas");
var stageWidth = canvasElement.width;
var stageHeight = canvasElement.height;
stage = new createjs.Stage(canvasElement);
stage.autoClear = false;
center.x = stageWidth / 2;
center.y = stageHeight / 2;
for(var i = 0; i < total; i++) {
var radius = 1 + Math.random() * 4;
var particle = new Particle(radius, "#0016E9");
resetParticle(particle);
particles.push(particle);
stage.addChild(particle);
}
addBackground(stageWidth, stageHeight, fading);
createjs.Ticker.timingMode = createjs.Ticker.RAF;
createjs.Ticker.addEventListener("tick", tick);
}
function resetParticle(particle) {
var radius = 1 + Math.random();
var angle = Math.random() * Math.PI * 2;
var lifetime = Math.random()* total | 0;
particle.reset(center.x, center.y, radius, angle, lifetime);
}
function tick() {
var count = particles.length;
for(var i = 0; i < count; i++) {
var particle = particles[i];
moveParticle(particle);
}
stage.update();
}
function moveParticle(particle) {
if (particle.lifetime > 0) {
particle.move(Math.PI / 90);
} else {
resetParticle(particle);
}
}
function addBackground(width, height, alpha) {
var background = new createjs.Shape();
background.graphics.beginFill("black")
.drawRect(0, 0, width, height)
.endFill();
stage.addChild(background);
background.alpha = alpha;
}
jsdo.