はじめに
前回に引き続いてBing APIをjQueryにより操作します。今回は画像や動画の検索です。
前回の掲載日
今回の内容とは関係がありませんが、
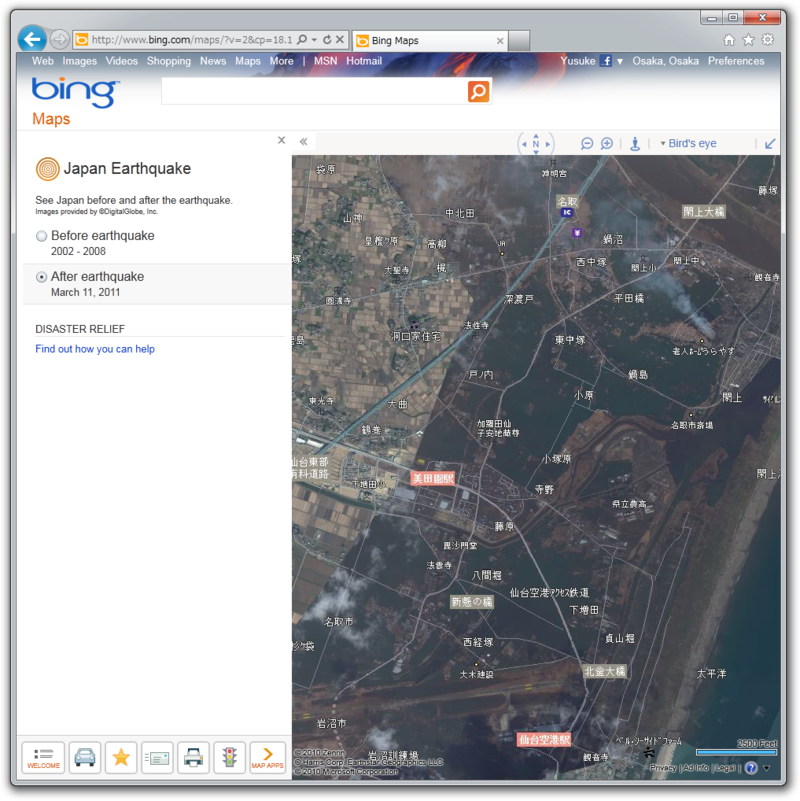
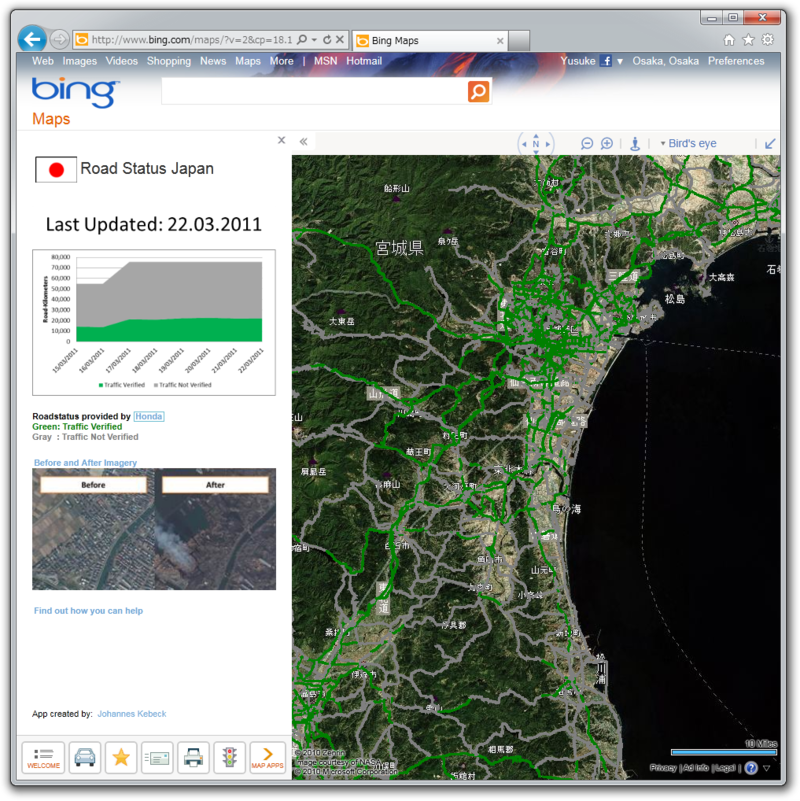
画像の検索
Bing APIで画像を検索してみましょう。APIの呼び出しは、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Image&
Image.Count=2&
Image.Offset=0
Image.
URLにアクセスすると次のようなJSON形式の結果が得られます。ひとつひとつの画像の結果は、
{
"SearchResponse": {
"Image": {
"Offset": 0,
"Results": [
{
"DisplayUrl": "http://windows.microsoft.com/lt-LT/windows7/what-is-windows-live",
"FileSize": 185115,
"Height": 441,
"MediaUrl": "http://res1.windows.microsoft.com/resbox/lt/Windows%207/main/3/1/31ded92b-cc47-41dd-a3ae-1eafaf199e92/31ded92b-cc47-41dd-a3ae-1eafaf199e92.jpg",
"Thumbnail": {
"ContentType": "image/jpeg",
"FileSize": 4692,
"Height": 160,
"Url": "http://ts1.mm.bing.net/images/thumbnail.aspx?q=809769770960&id=b7f7d82caa4e866d5a8838a832906dde",
"Width": 148
},
"Title": "Windows Live pagrindinis puslapis yra puiki vieta pradėti, kad ir ką ...",
"Url": "http://windows.microsoft.com/lt-LT/windows7/what-is-windows-live",
"Width": 410
},
{
"DisplayUrl": "http://windows.microsoft.com/nb-NO/windows-vista/Record-TV-in-Windows-Media-Center",
"FileSize": 150777,
"Height": 325,
"MediaUrl": "http://res1.windows.microsoft.com/resbox/nb/Windows%20Vista/Main/7/0/70d4ae6d-6866-437c-a466-5c908dc4ee57/70d4ae6d-6866-437c-a466-5c908dc4ee57.png",
"Thumbnail": {
"ContentType": "image/jpeg",
"FileSize": 3593,
"Height": 126,
"Url": "http://ts2.mm.bing.net/images/thumbnail.aspx?q=553917419693&id=ce43aa0c375b042edca53b0681ff9099",
"Width": 160
},
"Title": "Bla gjennom innspilt innhold i Windows Media Center",
"Url": "http://windows.microsoft.com/nb-NO/windows-vista/Record-TV-in-Windows-Media-Center",
"Width": 410
}
],
"Total": 186000
},
"Query": {
"SearchTerms": "Windows site:microsoft.com"
},
"Version": "2.0"
}
}
Image.
名前 | 説明 |
---|---|
FileSize | ファイルサイズ |
Title | タイトル |
MediaUrl | 画像へのURL |
Url | 画像を含むWebサイトへのURL |
DisplayUrl | 検索結果のページに表示するURL |
Width | 画像の横幅 |
Height | 画像の縦幅 |
ContentType | MIMEタイプ |
Thumbnail | サムネイルを表すThumbnailオブジェクト Url, ContentType, Width, Height, FileSizeプロパティを持つ |
コードの記述
それでは、
<!DOCTYPE html>
<html lang="ja">
<head>
<meta charset="utf-8" />
<title>Bing API Sample</title>
<link rel="stylesheet" href="default.css" type="text/css" />
<!-- jQuery ライブラリーの参照 -->
<script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.5.1.js" type="text/javascript"></script>
<!-- Bing API を利用する JavaScript ファイルの参照 -->
<script src="./iamge.js" type="text/javascript" charset="utf-8"></script>
</head>
<body>
<!-- 検索ボックス -->
<div id="box"><input type="text" id="query" /><input type="submit" id="search" value="" /></div>
<div style="clear: left;">
<!-- ここに検索の件数を表示する -->
<div id="summary"></div>
<!-- ここに検索結果のWebサイト情報を表示する -->
<ul id="list"></ul>
</div>
</body>
</html>
$(function () {
// AppId
var appId = "AppID";
// 特殊文字を置換する関数
var esc = function (str) {
if (!str) {
return "";
} else {
return str.replace(/&/g, "&").replace(/\</g, "<").replace(/\>/g, ">");
}
}
// (ここにコードを追記します)
});
検索ボックスのボタンをクリックしたときのAPI呼出し処理は次のようになります。前回のWebサイト検索と比べてBing APIのパラメーター部分が異なっています。
$("#search").click(function() {
var q = $("#query").val();
if (!q) {
// クエリーの入力がない場合は Bing へ移動
location.href = "http://www.bing.com/"
return;
}
// Bing API の非同期呼び出し
$.ajax({
type: "GET",
url: "http://api.bing.net/json.aspx",
dataType: "jsonp",
jsonp: "JsonCallback",
data: { // Bing API のパラメーター
AppId: AppId,
Version: "2.2",
Market: "ja-JP",
Query: q,
Sources: "Image",
"Image.Count": 10,
"Image.Offset": 0,
JsonType: "callback" // ← JSONP 形式の結果を指定
},
success: function (data) {
// 非同期呼出しが成功した場合
searchCallback(data);
},
error: function (jqXHR, textStatus, errorThrown) {
// 非同期呼出しに失敗した場合
alert(errorThrown);
}
});
});
続いてレスポンスの処理は次の通りです。上記コードの前に記述します。
var searchCallback = function (response) {
// 結果がエラーの場合
// (省略 第19回参照)
var list = $("#list");
list.empty();
var summary = $("#summary");
summary.empty();
if (response &&
response.SearchResponse &&
response.SearchResponse.Image &&
response.SearchResponse.Image.Results) {
// 結果の一覧表示
$.each(response.SearchResponse.Image.Results, function () {
list.append('<li><a href="' + this.Url + '">' +
'<img src="' + this.Thumbnail.Url + '" width="' + this.Thumbnail.Width + '" height="' + this.Thumbnail.Height + '" title="' + esc(this.Title) + '" /></a><br />' +
'<span class="dispUrl">' + esc(this.DisplayUrl) + '</span></li>');
});
// 件数の表示
var offset = response.SearchResponse.Web.Offset;
summary.html((offset + 1) + "-" + (offset + response.SearchResponse.Image.Results.length) + " 件 " +
"(" + response.SearchResponse.Web.Total + "件中) の検索結果");
} else {
list.append("<li>No results</li>");
}
}
以上の実行結果は図4のようになります。正しく動作したでしょうか。
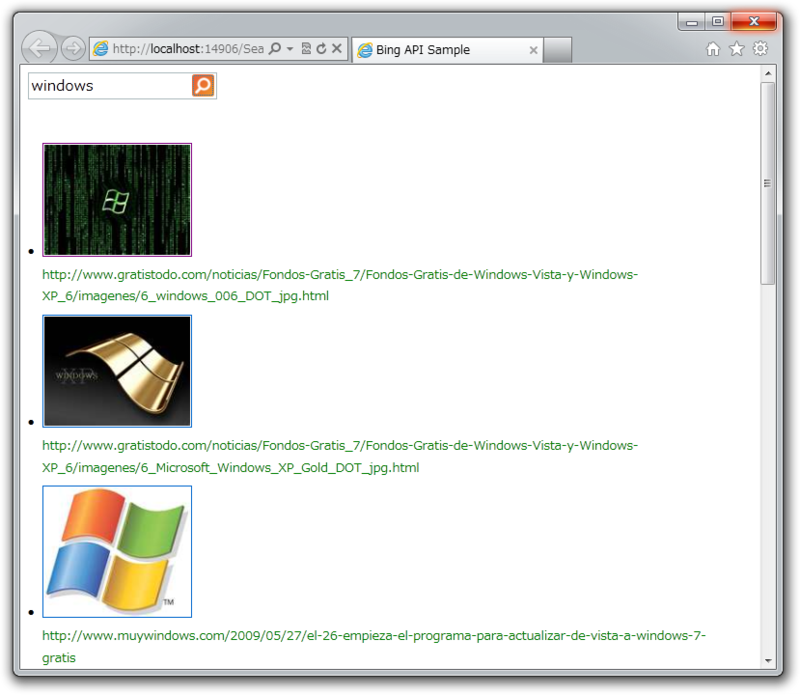
フィルタリング
さて、
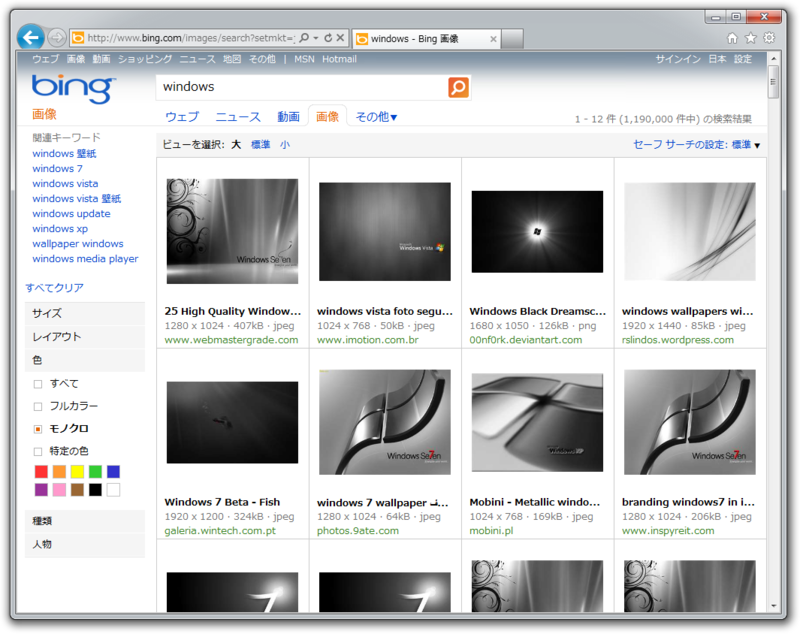
フィルタリングの指定は、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Image&
Image.Count=2&
Image.Offset=0&
Image.Filters=Size:Samll
指定可能な値はMSDN Libraryを参照してください。複数のフィルタリング項目の指定も可能です。その場合は空白で区切って指定します。
jQueryの非同期呼出し部分に直接指定した場合は次のようになります。
$.ajax({
type: "GET",
url: "http://api.bing.net/json.aspx",
dataType: "jsonp",
jsonp: "JsonCallback",
data: { // Bing API のパラメーター
AppId: AppId,
Version: "2.2",
Market: "ja-JP",
Query: q,
Sources: "Image",
"Image.Count": 10,
"Image.Offset": 0,
"Image.Filters": "Size:Large Color:Monochrome", // フィルタリング項目の指定
JsonType: "callback"
},
success: function (data) { /* (省略) */ },
error: function (jqXHR, textStatus, errorThrown) { /* (省略) */ }
});
また、
動画の検索
次は動画の検索をしてみましょう。APIの呼び出しは次のようなURLにアクセスします。Sourcesパラメーターの値がVideo、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Video&
Video.Count=2&
Video.Offset=0
Video.
URLにアクセスすると次のようなJSON形式の結果が得られます。動画検索の場合、
{
"SearchResponse": {
"Query": {
"SearchTerms": "Windows"
},
"Version": "2.2",
"Video": {
"Offset": 0,
"Results": [
{
"ClickThroughPageUrl": "http://www.bing.com/videos/search?q=Windows&scope=video&docid=863516491784&FORM=SOAPGN",
"PlayUrl": "http://video.ap.org/?f=AP&pid=HqbGANuCLP0Oc_cWJlaVHQths7NMIpj0",
"RunTime": 118282,
"SourceTitle": "AP",
"StaticThumbnail": {
"ContentType": "image/jpeg",
"FileSize": 3915,
"Height": 94,
"Url": "http://ts1.mm.bing.net/videos/thumbnail.aspx?q=863516491784&id=41bd8c6dd60ad9d158bbe4d8d3e7a534&bid=mhpnR5m4E0jRqQ&bn=Thumb&url=http%3a%2f%2fvideo.ap.org%2f%3ff%3dAP%26pid%3dHqbGANuCLP0Oc_cWJlaVHQths7NMIpj0",
"Width": 160
},
"Title": "Raw Video: Protest in London Against Cuts"
},
{
"ClickThroughPageUrl": "http://www.bing.com/videos/search?q=Windows&scope=video&docid=527796928524&FORM=SOAPGN",
"PlayUrl": "http://www.5min.com/Video/Windows-7-Touch-Screen-Features-Review-239390015",
"RunTime": 199000,
"SourceTitle": "5min",
"StaticThumbnail": {
"ContentType": "image/jpeg",
"FileSize": 3235,
"Height": 88,
"Url": "http://ts1.mm.bing.net/videos/thumbnail.aspx?q=527796928524&id=3ec8e77088f94e3085b096703366c2cb&bid=rZJuqRjbwPakeg&bn=Thumb&url=http%3a%2f%2fwww.5min.com%2fVideo%2fWindows-7-Touch-Screen-Features-Review-239390015",
"Width": 160
},
"Title": "Windows 7 Touch Screen Features Review"
}
],
"Total": 606000
}
}
}
Video.
名前 | 説明 |
---|---|
Title | タイトル |
SourceTitle | 動画サイトの名前 |
RunTime | 動画の長さ |
PlayUrl | 動画へのURL |
ClickThroughPageUrl | Bingの動画ページのURL |
StaticThumbnail | 動画のサムネイルを表すThumbnailオブジェクト |
コードの記述
jQueryによるAPIの呼び出しや、
APIの呼び出し部分は次のようになります。
$.ajax({
type: "GET",
url: "http://api.bing.net/json.aspx",
dataType: "jsonp",
jsonp: "JsonCallback",
data: { // Bing API のパラメーター
AppId: AppId,
Version: "2.2",
Market: "ja-JP",
Query: q,
Sources: "Video",
"Video.Count": 10,
"Video.Offset": 0,
JsonType: "callback"
},
success: function (data) { /* (省略) */ },
error: function (jqXHR, textStatus, errorThrown) { /* (省略) */ }
});
レスポンスの処理は次の通りです。
var searchCallback = function (response) {
// 結果がエラーの場合
// (省略 第19回参照)
var list = $("#list");
list.empty();
var summary = $("#summary");
summary.empty();
if (response &&
response.SearchResponse &&
response.SearchResponse.Video &&
response.SearchResponse.Video.Results) {
// 結果の一覧表示
$.each(response.SearchResponse.Video.Results, function () {
list.append('<li><a href="' + this.PlayUrl + '">' +
'<img src="' + this.StaticThumbnail.Url + '" width="' + this.StaticThumbnail.Width + '" height="' + this.StaticThumbnail.Height + '" alt="' + esc(this.Title) + '" /></a><br />' +
'<span class="sourceTitle">' + esc(this.SourceTitle) + '</span><br />' +
'<span class="runTime">' + runTimeFormat(this.RunTime) + '</span></li>');
});
// 件数の表示
var offset = response.SearchResponse.Video.Offset;
summary.html((offset + 1) + "-" + (offset + response.SearchResponse.Video.Results.length) + " 件 " +
"(" + response.SearchResponse.Video.Total + "件中) の検索結果");
} else {
list.append("<li>No results</li>");
}
}
動画の長さ
// ミリ秒を 0:00 形式に変換する関数
var runTimeFormat = function(runTime) {
if (!runTime) {
return "";
} else {
var t = runTime / 1000;
return Math.floor(t / 60) + ":" + ("0" + t % 60).slice(-2);
}
}
動画サイト名と動画の長さ用のスタイルは前回定義していなかったので、
.sourceTitle {
font-size: small;
color: Green;
}
.runTime {
font-size: small;
color: Gray;
}
以上を実行すると図5のようになります。
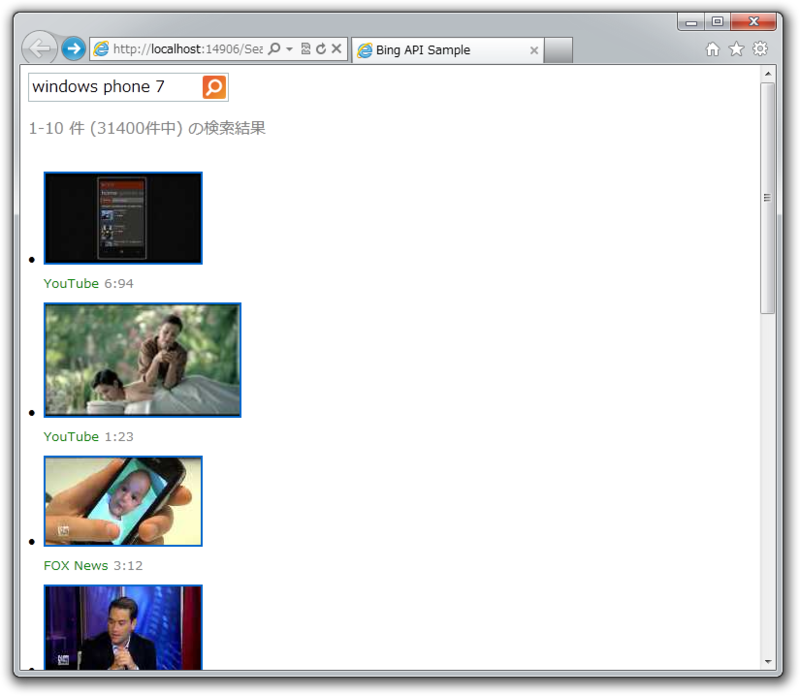
フィルタリング
Bingの動画検索を見てみると、
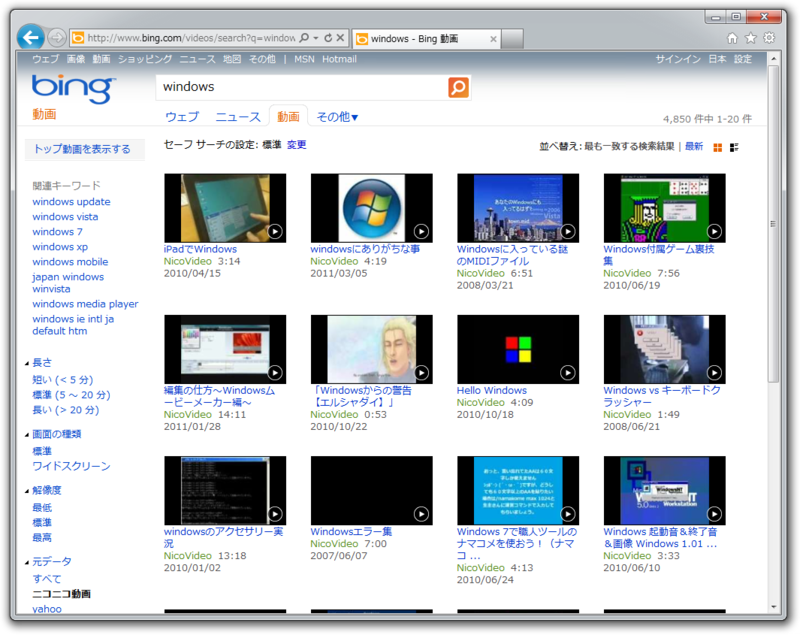
Bingの動画検索では、
APIでも利用できる内容は、
- http://
api. bing. net/ json. aspx?
AppId=AppId&
Version=2.2 &
Market=ja-JP&
Query=Windows&
Sources=Video&
Video.Count=2&
Video.Offset=0&
Video.Filters=Duration:Short&
Video.SortBy=Date
Video.
jQueryの非同期呼出し部分に直接指定した場合は次のようになります。
$.ajax({
type: "GET",
url: "http://api.bing.net/json.aspx",
dataType: "jsonp",
jsonp: "JsonCallback",
data: { // Bing API のパラメーター
AppId: AppId,
Version: "2.2",
Market: "ja-JP",
Query: q,
Sources: "Video",
"Video.Count": 10,
"Video.Offset": 0,
"Video.Filters": "Duration:Short Aspect:Standard", // フィルタリング項目の指定
"Video.SortBy": "Date", // または "Relevance" が指定可
JsonType: "callback"
},
success: function (data) { /* (省略) */ },
error: function (jqXHR, textStatus, errorThrown) { /* (省略) */ }
});
フィルタリングの指定は、
今回はここまでです。いかがでしたでしょうか。次回は、