こんにちは、
前回はcanvas、
Chrome拡張で使えるECMAScript 5
Google ChromeのJavaScriptエンジンはGoogle製のV8ですが、
Native JSON
まず、
JavaScriptにおけるJSONのメソッドは、
var data = {
a:1,
b:[1,2],
c:new Date(),
f:function(a){return a;}
};
var json_text = JSON.stringify(data);
console.log(json_text);
//{"a":1,"b":[1,2],"c":"2010-01-18T14:01:36Z"}
var json_text2 = JSON.stringify(data,null,2);
console.log(json_text2);
/*
{
"a": 1,
"b": [
1,
2
],
"c": "2010-01-18T14:01:36Z"
}
*/
JSON.
function replacer(key, value) {
if (this[key] instanceof Date) {
return this[key].getTime();
} else if (this[key] instanceof Function) {
return this[key].toString();
} else {
return value;
}
}
var json_text3 = JSON.stringify(data, replacer,'\t');
console.log(json_text3);
/*
{
"a": 1,
"b": [
1,
2
],
"c": 1263823296890,
"f": "function (a){return a;}"
}
*/
var data2 = JSON.parse(json_text);
console.log(data2);
JSON.
また、
JSON.
なお、
Array拡張
配列メソッドとしてindexOf, lastIndexOf, every, some, forEach, map, filter, reduce, reduceRightが追加されています。indexOf, lastIndexOfは配列を検索し、
var list = [1,2,3];
list.forEach(function(item, i, array){
console.log(item);
});
このArray拡張はFirefoxでは1.
Object.keys
Object.
var data = {
a:1,
b:[1,2],
c:new Date(),
f:function(a){return a;}
};
console.log(Object.keys(data));
//["a", "b", "c", "f"]
そのオブジェクト自身が持つプロパティのみを列挙し、
function Point(x, y){
this.x = x;
this.y = y;
}
Point.prototype = {
clone:function(){
return new Point(this.x, this.y);
}
};
var p1 = new Point(1, 2);
console.log(Object.keys(p1));
// ["y", "x"]
Object.
if (!Object.keys){
Object.keys = function(object){
if (!(object instanceof Object)) {
throw new TypeError('Object.keys called on non-object')
}
var keys = [];
for (var key in object){
if(object.hasOwnProperty(key)){
keys.push(key);
}
}
return keys;
}
}
console.log(Object.keys(data));
//["a", "b", "c", "f"]
なお、
Object.create
※2010年1月にリリースされたGoogle Chrome 4.
Object.
var Point = {
clone:function(){
return new Point(this.x, this.y);
}
};
var p1 = Object.create(Point,{
x:{
value: 1,
writable: true,
enumerable: true,
configurable: true
},
y:{
value:2,
writable: true,
enumerable: true,
configurable: true
}
});
console.log(p1);
// ["y", "x"]
Object.
Object.
CSS3
Google Chromeは最新のWebKitを採用していますので、
border-radius
border-radiusはいわゆる角丸を実現します。WebKitでは-webkit-border-radiusのようにプリフィックスが付きます。将来的にはこのプリフィックスは不要になる点に注意が必要です。実際、
#box{
border:3px solid #0099cc;
border-radius:15px;
}
gradient
gradientは背景などでグラデーションを実現します。
#box{
background: -webkit-gradient(linear, left top, left bottom,
from(#99cccc), to(#3399cc));
width:100px;
height:100px;
}
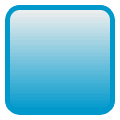
CSSの応用
下記のようなCSSでボタンにスタイルを当てると、
input[type="button"],
input[type="submit"],
button{
-webkit-appearance: button;
color:#ffffff;
background: -webkit-gradient(linear, left top, left bottom,
from(#777777), to(#333333));
border: 1px solid #999999;
border-radius: 3px;
height: 1.7em;
padding: 0 0.6em;
vertical-align: middle;
}
input[type="button"]:hover,
input[type="submit"]:hover,
button:hover{
background: -webkit-gradient(linear, left top, left bottom,
from(#999999), to(#666666));
}
input[type="button"][disabled],
input[type="submit"][disabled],
button[disabled]{
background:#999999;
}
input[type="button"][disabled]:hover,
input[type="submit"][disabled]:hover,
button[disabled]:hover{
background:#999999;
}

まとめ
今回はChrome拡張で使えるHTML5周辺技術としてECMAScript 5とCSS3を取り上げました。次回はさらにHTML5周辺技術から、