はじめに
今回はJavaScriptライブラリーで提供されている地図コントロール、
また、
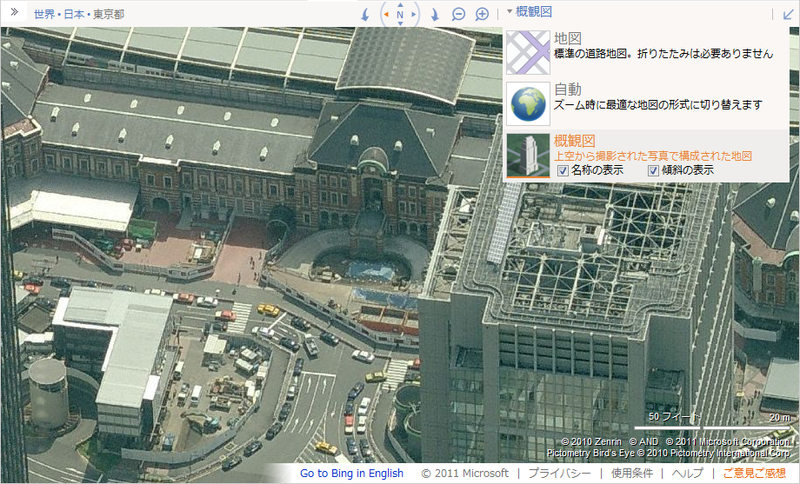
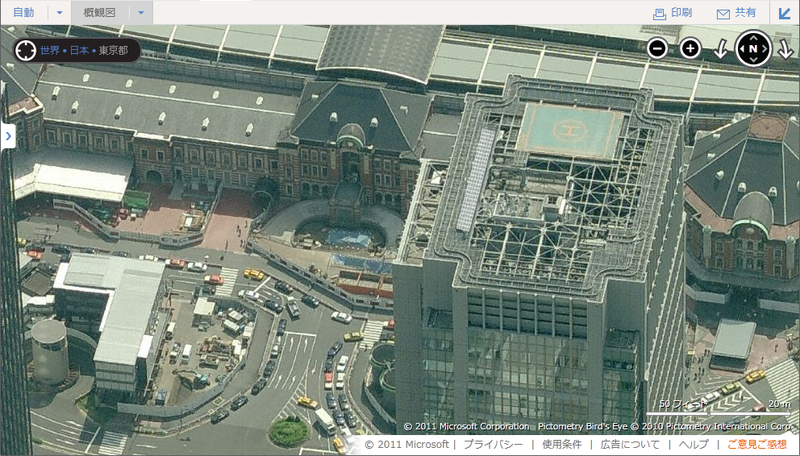
さて、
- ルート検索
(経路検索) および表示 (図3) - 交通情報の表示
(図4) - ショッピングモールなどの地図表示と情報の取得
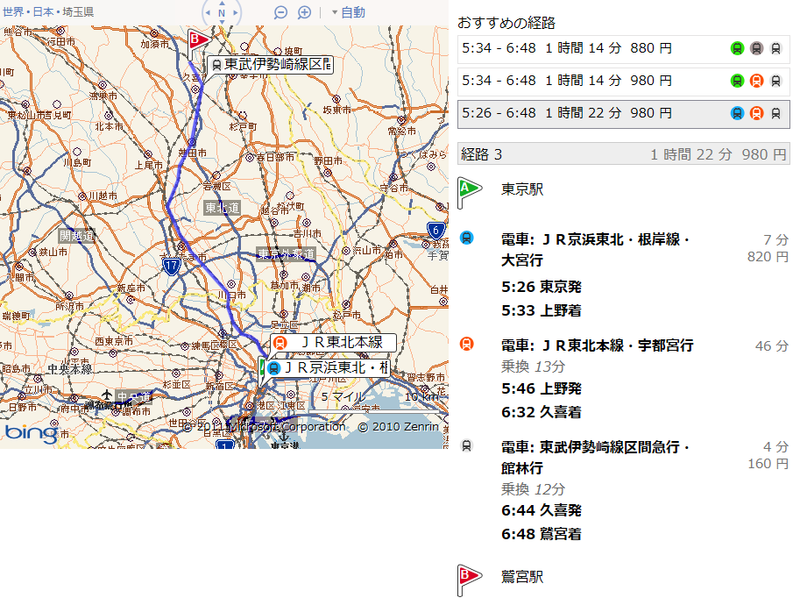
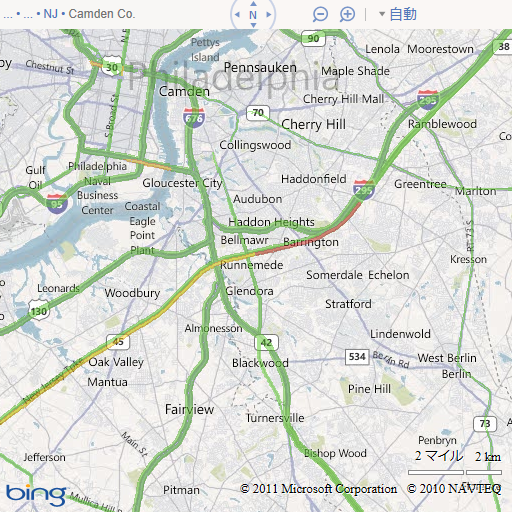
以上の機能は追加モジュールとして参照し使用します。今回はこれらの機能を順番に紹介します。
モジュールの登録とロード
最初に、
モジュールの作成
さっそく使ってみましょう。モジュールとして登録するスクリプトを作成します。内容はなんでも構いませんが、
function MyModule(map) {
this.drawCircle = function(location, meters) {
// (省略)
}
}
Microsoft.Maps.moduleLoaded("GihyoSample.MyModule");
スクリプトで使用する関数や、
モジュールの登録
モジュールを作成した次は、
Microsoft.Maps.registerModule(
"GihyoSample.MyModule",
"http://example.jp/MyModule.js");
registerModuleメソッドの引数は、
オプションとして、
Microsoft.Maps.registerModule(
"GihyoSample.MyModule",
"http://example.jp/MyModule.js",
{styleUrls: ["http://example.jp/MyModule.css"]});
モジュールのロード
最後にモジュールのロードです。モジュールを使用するときは、
Microsoft.Maps.loadModule(
"GihyoSample.MyModule",
{callback: moduleLoaded});
モジュールのロードが完了すると、
サンプル
以上をまとめたサンプルを示します。次のコード中では、
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Module Sample</title>
<script type="text/javascript" src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=7.0&mkt=ja-jp"></script>
<script type="text/javascript">
var map = null;
function ModuleLoaded()
{
var loc = new Microsoft.Maps.Location(35.55, 139.7862);
map.setView({center: loc, zoom: 12});
// モジュールの利用
var module = new MyModule(map);
module.drawCircle(loc, 5000);
}
function GetMap()
{
// 地図の表示
var options = {credentials: "BingMapsKey"};
map = new Microsoft.Maps.Map(document.getElementById("map"), options);
// モジュールの登録とロード
Microsoft.Maps.registerModule("GihyoSample.MyModule", "MyModule.js");
Microsoft.Maps.loadModule("GihyoSample.MyModule", {callback: ModuleLoaded});
}
</script>
</head>
<body onload="GetMap();">
<div id="map" style="position:relative;width:512px;height:512px;"></div>
</body>
</html>
実行結果は図5のようになります。
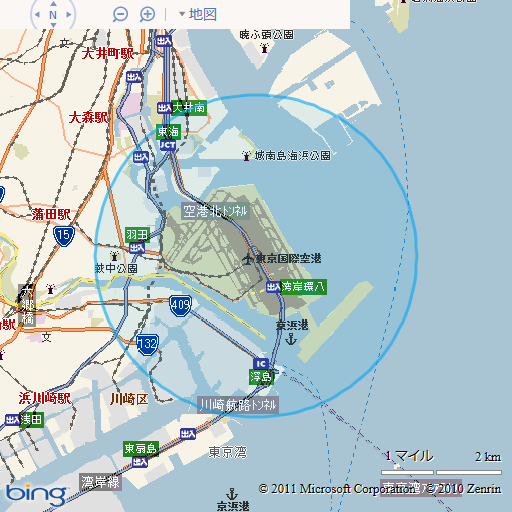
ルート検索
続いてBing Maps AJAX Controlの新しい機能をみていきましょう。ルート検索は、
新しい機能を利用すれば、
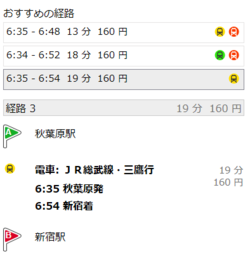
もちろん地図コントロール内にもルート検索結果の表示や、
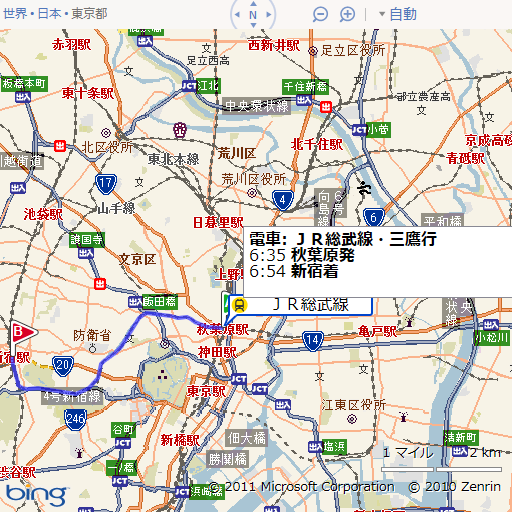
ルート検索の種類は次の3種類が提供されています。
- 自動車のルート探索
(ドライブルート) - 徒歩のルート検索
- 電車・
バスなどのルート検索 (乗り換え案内)
これらのルート探索は、
<script type="text/javascript" src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=7.0&mkt=ja-jp"></script>
乗り換え案内は、
コードの記述
それでは、
HTMLファイルは次のようになります。<body>要素内には、
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Direction Sample</title>
<script type="text/javascript" src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=7.0&mkt=ja-jp"></script>
<script type="text/javascript">
var map = null;
function GetMap()
{
var options = {credentials: "BingMapsKey", showBreadcrumb: true};
map = new Microsoft.Maps.Map(document.getElementById("map"), options);
Microsoft.Maps.loadModule("Microsoft.Maps.Directions", {callback: ModuleLoaded});
}
function ModuleLoaded()
{
// (ここにルート検索処理を追記します)
}
</script>
</head>
<body onload="GetMap();">
<div id="map" style="position:relative;width:512px;height:512px;float:left;"></div>
<div id="itinerary" style="position:relative;width:400px;float:left;"></div>
</body>
</html>
GetMap関数内で、
Microsoft.Maps.loadModule("Microsoft.Maps.Directions", {callback: ModuleLoaded});
次にモジュールをロードした後に呼ばれるModuleLoaded関数の処理を追記します。
ルート検索処理は、
function ModuleLoaded()
{
// DirectionsManager オブジェクトの生成 (Map オブジェクトを渡す)
var directionsManager = new Microsoft.Maps.Directions.DirectionsManager(map);
// 出発・到着地点を追加
var startWaypoint = new Microsoft.Maps.Directions.Waypoint({address: "東京"});
var endWaypoint = new Microsoft.Maps.Directions.Waypoint({address: "大阪"});
directionsManager.addWaypoint(startWaypoint);
directionsManager.addWaypoint(endWaypoint);
// UI の描画 (<div>要素の id 値を指定)
directionsManager.setRenderOptions({itineraryContainer: document.getElementById("itinerary")});
// 検索オプションの指定
// (routeMode は driving, transit, walking のいずれかを指定)
// (timeType は arrival, departure, lastAvailable のいずれかを指定)
directionsManager.setRequestOptions({
routeMode: Microsoft.Maps.Directions.RouteMode.transit,
transitOptions: {
timeType: Microsoft.Maps.Directions.TimeType.departure,
transitTime: new Date}});
// イベント処理 (ここではルート検索時のエラーイベントを処理)
Microsoft.Maps.Events.addHandler(directionsManager, "directionsError", function (e) {
alert("responseCode: " + e.responseCode + "\n" + e.message);
});
// ルート検索 (結果を地図に表示)
directionsManager.calculateDirections();
}
上記コードの実行結果は図8のようになります。
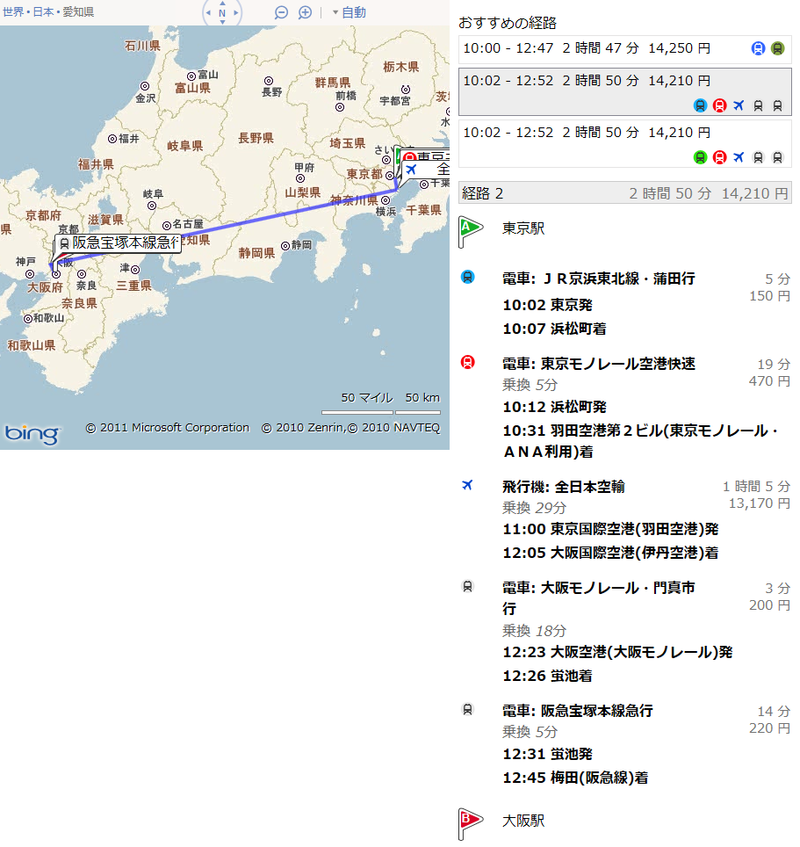
ルート検索は、
ドライブルートの結果も示しておきます。次のコードでは、
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Direction Sample</title>
<script type="text/javascript" src="http://ecn.dev.virtualearth.net/mapcontrol/mapcontrol.ashx?v=7.0&mkt=en-us"></script>
<script type="text/javascript">
var map = null;
function GetMap()
{
var options = {credentials: "BingMapsKey", showBreadcrumb: true};
map = new Microsoft.Maps.Map(document.getElementById("map"), options);
Microsoft.Maps.loadModule("Microsoft.Maps.Directions", {callback: ModuleLoaded});
}
function ModuleLoaded()
{
// DirectionsManager オブジェクトの生成 (Map オブジェクトを渡す)
var directionsManager = new Microsoft.Maps.Directions.DirectionsManager(map);
// 出発・到着地点を追加
var startWaypoint = new Microsoft.Maps.Directions.Waypoint({address: "Seattle, WA"});
var endWaypoint = new Microsoft.Maps.Directions.Waypoint({address: "Portland, OR"});
directionsManager.addWaypoint(startWaypoint);
directionsManager.addWaypoint(endWaypoint);
// UI の描画 (<div>要素の id 値を指定)
directionsManager.setRenderOptions({itineraryContainer: document.getElementById("itinerary")});
// ルート検索 (結果を地図に表示)
directionsManager.calculateDirections();
}
</script>
</head>
<body onload="GetMap();">
<div id="map" style="position:relative;width:512px;height:512px;float:left;"></div>
<div id="itinerary" style="position:relative;width:400px;float:left;"></div>
</body>
</html>
実行結果は図9のようになります。ドライブや徒歩ルートの場合、
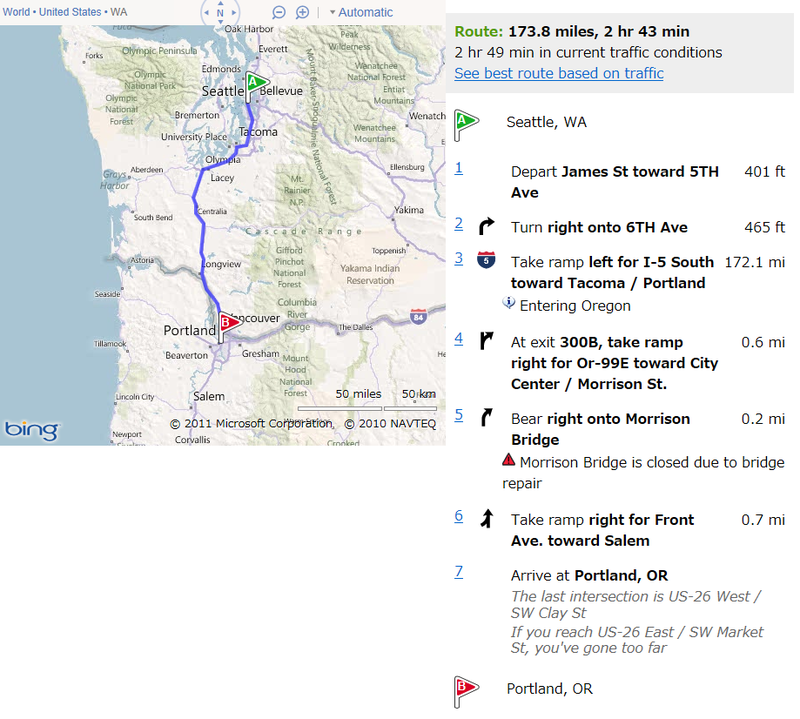
交通情報の表示
次は交通情報の表示です。渋滞情報を視覚的に表示します
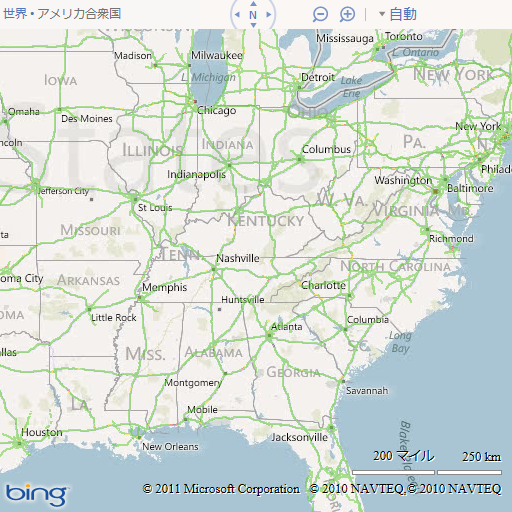
交通情報の表示機能はシンプルです。さっそくコードを書いてみましょう。モジュールのキー名は、
Microsoft.Maps.loadModule("Microsoft.Maps.Traffic", {callback: ModuleLoaded});
モジュールをロードすると、
function ModuleLoaded()
{
var trafficLayer = new Microsoft.Maps.Traffic.TrafficLayer(map);
trafficLayer.show();
}
交通情報の表示は、
交通情報のタイル情報は、
あまり利用することはないと思いますが、
alert(trafficLayer.getTileLayer().getTileSource("mercator").getUriConstructor());
また、
var tileLayer = trafficLayer.getTileLayer();
tileLayer.setOptions({opacity: 0.5});
屋内の地図の表示
次は、
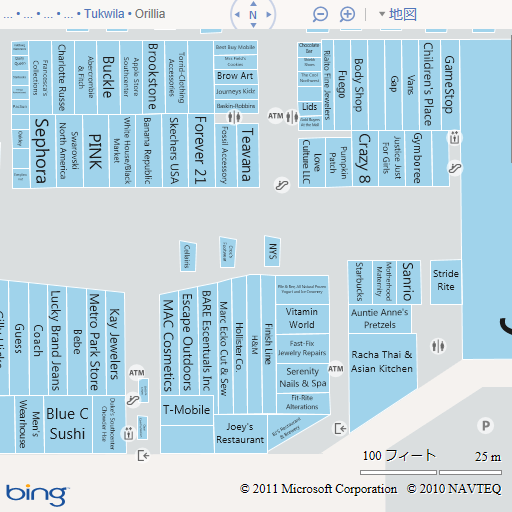
今のところ、
屋内の地図情報を得るには、
- IDの指定
- 指定した地点付近から検索
各地図にはIDが割り当てられており、
コードの記述
それではコードを書いていきましょう。モジュールのキー名は、
Microsoft.Maps.loadModule("Microsoft.Maps.VenueMaps", {callback: ModuleLoaded});
屋内の地図情報を得るには、
IDの指定
まず、
function ModuleLoaded()
{
var factory = new Microsoft.Maps.VenueMaps.VenueMapFactory(map);
factory.create({venueMapId: "hcl-seatacairportd",
success: ShowVenue,
error: ShowError});
}
createメソッドに渡すオプションは次の通りです。
名前 | 説明 |
---|---|
venueMapId | 屋内地図のID |
success | 結果を受け取る関数を指定 |
error | エラー発生時の関数を指定 |
結果は、
function ShowVenue(venue)
{
map.setView(venue.bestMapView);
venue.show();
}
function ShowError(errorCode)
{
alert("Error Code: " + errorCode);
}
VenueMapクラスのbestMapViewプロパティには、
ここまでの内容を実行した結果は図12、
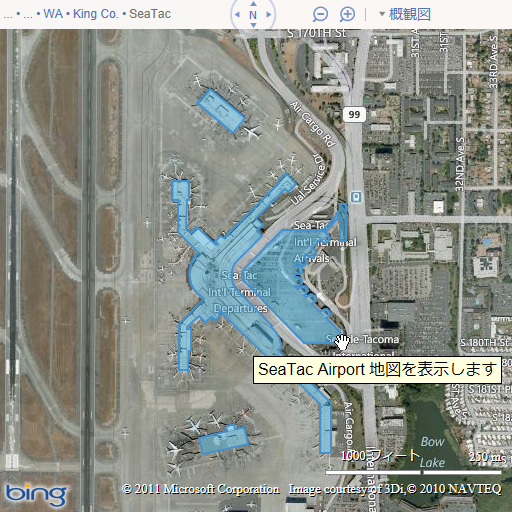
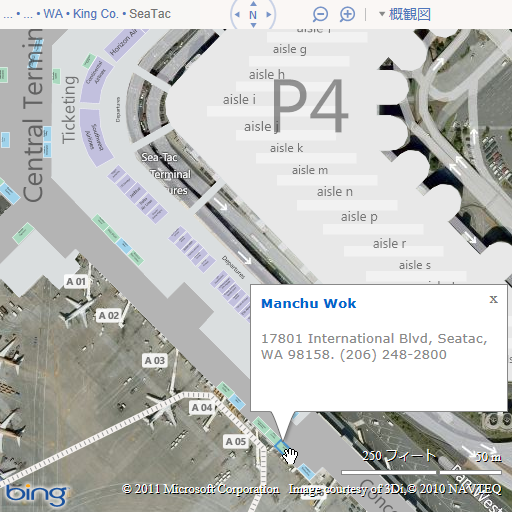
指定した地点付近から検索
次に経緯度と半径を指定し、
function ModuleLoaded()
{
var factory = new Microsoft.Maps.VenueMaps.VenueMapFactory(map);
factory.getNearbyVenues(
{map: map,
location: map.getCenter(),
radius: 20000, // 20km
callback: DisplayNearbyVenueCount});
}
getNearbyVenuesメソッドに渡すオプションは次の通りです。
名前 | 説明 |
---|---|
map | Mapオブジェクト |
location | 検索する地点の経緯度 |
radius | 検索範囲の半径 |
callback | 検索結果を受け取る関数を指定 |
オプションのradiusに、
プロパティ | 説明 |
---|---|
distance | 指定した地点からの距離 |
metadata | IDなどの地図情報 |
検索結果を受け取るDisplayNearbyVenueCount関数の内容を記述します。ここでは指定した地点からの距離と、
function DisplayNearbyVenueCount(venues)
{
var displayResults = "";
for (var i = 0; i < venues.length; i++) {
displayResults += venues[i].metadata.Name +
"\n\tID: " + venues[i].metadata.MapId +
"\n\tDistance: " + (venues[i].distance / 1000).toFixed(1) + " km\n";
}
alert(displayResults);
}
実行結果は図14のようにメッセージが表示されます。
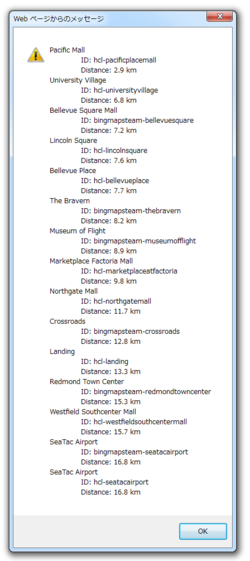
この屋内の地図表示に関して、
おわりに
今回はここまでです。いかがでしたでしょうか。乗り換え案内以外は、
また、
第26回のガソリンスタンドアプリのサンプルも公開していますので、